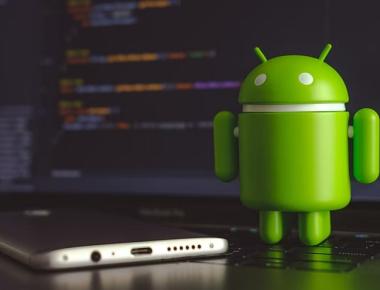
React Native is a popular JavaScript library for building mobile applications, and QR codes are increasingly becoming an essential part of mobile apps. React Native QRCode SVG is a library that makes it easy to create and display QR codes in React Native applications using Scalable Vector Graphics (SVG). In this blog, we will explore the features of React Native QRCode SVG and how to use it to create QR codes in React Native applications.
React Native QRCode SVG is a powerful library that makes it easy to create and display QR codes in React Native applications. Its features, including SVG rendering, customizable options, and error correction, make it a must-have for any developer looking to implement QR codes in their mobile apps. With this library, you can generate and customize QR codes to meet your specific needs and enhance the user experience of your application.
React Native QRCode SVG is a lightweight and easy-to-use library that offers several features for creating and displaying QR codes in React Native applications. Some of the notable features include:
npm install react-native-qrcode-svg
and
npm install react-native-svg
import React, { useState } from 'react'; import { SafeAreaView, StyleSheet, TextInput, View, } from 'react-native'; import QRCode from 'react-native-qrcode-svg'; const App = () => { const [url, setUrl] = useState(''); const handleTextChange = (text) => { setUrl(text); }; return ( <SafeAreaView style={styles.container}> <View style={styles.inputContainer}> <TextInput style={styles.input} placeholder="Enter URL" onChangeText={handleTextChange} value={url} /> </View> {url ? ( <View style={styles.qrCodeContainer}> <QRCode value={url} size={200} color="black" backgroundColor="white" /> </View> ) : null} </SafeAreaView> ); }; const styles = StyleSheet.create({ container: { flex: 1, alignItems: 'center', justifyContent: 'center', backgroundColor: '#F5FCFF', }, inputContainer: { width: '90%', backgroundColor: '#FFFFFF', borderRadius: 5, paddingHorizontal: 10, paddingVertical: 5, marginBottom: 20, shadowColor: '#000000', shadowOpacity: 0.3, shadowRadius: 2, shadowOffset: { height: 1, width: 0.3, }, elevation: 2, }, input: { fontSize: 16, }, qrCodeContainer: { backgroundColor: '#FFFFFF', borderRadius: 5, paddingHorizontal: 10, paddingVertical: 10, shadowColor: '#000000', shadowOpacity: 0.3, shadowRadius: 2, shadowOffset: { height: 1, width: 0.3, }, elevation: 2, }, }); export default App;
Coming Soon…
Quick Links
Legal Stuff