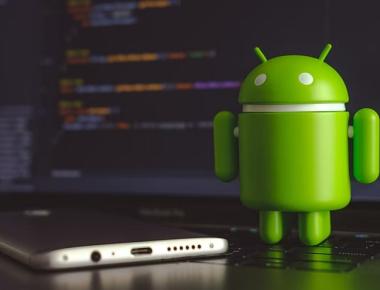
Exploring Alternative Emulators for React Native Development
January 20, 2024
1 min
Using bare setTimeout, setInterval, setImmediate and requestAnimationFrame calls is very dangerous because if you forget to cancel the request before the component is unmounted, you risk the callback throwing an exception.
If you include TimerMixin, then you can replace your calls to setTimeout(fn, 500) with this.setTimeout(fn, 500) (just prepend this.) and everything will be properly cleaned up for you.
npm install react-timer-mixin
import React, { Component } from 'react'; import { View, Text, StyleSheet } from 'react-native'; import TimerMixin from 'react-timer-mixin'; export default class App extends Component { constructor(props) { super(props); this.state = { countdown: 10, // set the initial countdown time in seconds }; } componentDidMount() { this.timer = TimerMixin.setInterval(() => { if (this.state.countdown === 0) { TimerMixin.clearInterval(this.timer); return; } this.setState((prevState) => ({ countdown: prevState.countdown - 1, })); }, 1000); } componentWillUnmount() { TimerMixin.clearInterval(this.timer); } render() { return ( <View style={styles.container}> <Text style={styles.timerText}>{this.state.countdown}</Text> </View> ); } } const styles = StyleSheet.create({ container: { flex: 1, justifyContent: 'center', alignItems: 'center', }, timerText: { fontSize: 48, }, });
Coming Soon…
Quick Links
Legal Stuff