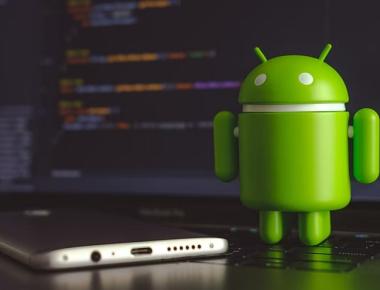
Exploring Alternative Emulators for React Native Development
January 20, 2024
1 min
setGestureState
Boolean
false
. true
onPanBegin({ originX, originY })
Function
onPan({ absoluteChangeX, absoluteChangeY, changeX, changeY })
Function
onPanEnd()
Function
panDecoratorStyle
Object
resetPan
Boolean
true
is passed, it will reset the state of the panning decorator. This can be useful if you want to reset the absolute change values, since these stay stored until you reset them.setGestureState
Boolean
false
.horizontal
Boolean
false
vertical
Boolean
false
left
Boolean
false
right
Boolean
false
up
Boolean
false
up
Boolean
false
continuous
Boolean
true
, then you will receive an update each time the touch moves. If false
you will only receive a single notification about the touch. true
initialVelocityThreshold
Number
0.7
verticalThreshold
Number
10
horizontalThreshold
Number
10
onSwipeBegin({ direction, distance, velocity })
Function
onSwipe({ direction, distance, velocity })
Function
continuous
is true
.onSwipeEnd({ direction })
Function
swipeDecoratorStyle
Object
npm i react-native-gesture-recognizers
import React, { useRef } from 'react'; import { View, Image, StyleSheet } from 'react-native'; import { PanGestureHandler } from 'react-native-gesture-handler'; export default function App() { const panRef = useRef(null); const imageRef = useRef(null); const onPanGestureEvent = ({ nativeEvent }) => { imageRef.current.setNativeProps({ style: { transform: [ { translateX: nativeEvent.translationX }, { translateY: nativeEvent.translationY }, ], }, }); }; return ( <View style={styles.container}> <PanGestureHandler ref={panRef} onGestureEvent={onPanGestureEvent} minDist={10} > <Image ref={imageRef} source={{ uri: 'https://c4.wallpaperflare.com/wallpaper/540/1009/255/meme-lol-face-wallpaper-preview.jpg' }} style={styles.image} /> </PanGestureHandler> </View> ); } const styles = StyleSheet.create({ container: { flex: 1, justifyContent: 'center', alignItems: 'center', backgroundColor: '#F5FCFF', }, image: { width: 200, height: 200, }, });
Coming Soon…
Quick Links
Legal Stuff