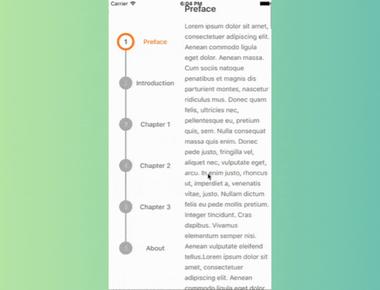
React Native Multi-Slider is a customizable and easy-to-use component that allows users to select a range of values by sliding between minimum and maximum values. It is built using React Native and is compatible with both iOS and Android platforms. The multi-slider is widely used in mobile applications to allow users to set ranges such as price ranges, date ranges, or any other numeric range selection. React Native Multi-Slider supports several customization options such as setting the minimum and maximum values, step value, track and thumb colors, and more. It also includes features such as displaying labels for the start and end values, displaying the selected range in real-time, and handling touch events for precise selection. One of the significant advantages of using React Native Multi-Slider is that it is highly customizable and can be easily integrated into any React Native project. It also provides a great user experience and can make your app more user-friendly by providing a simple and intuitive way for users to select ranges. Overall, React Native Multi-Slider is a great component to use in your mobile applications when you need to allow users to select a range of values. Its ease of use and customizability make it a popular choice among React Native developers.
npm install @ptomasroos/react-native-multi-slider
Or
yarn add @ptomasroos/react-native-multi-slider
import React, { useState } from 'react'; import { StyleSheet, View, Text } from 'react-native'; import MultiSlider from '@ptomasroos/react-native-multi-slider'; const App = () => { const [simpleValue, setSimpleValue] = useState(0); const [twoWayValue, setTwoWayValue] = useState([10, 30]); const [customValue, setCustomValue] = useState([20, 40, 60]); const handleSimpleSliderChange = value => { setSimpleValue(value); }; const handleTwoWaySliderChange = value => { setTwoWayValue(value); }; const handleCustomSliderChange = value => { setCustomValue(value); }; return ( <View style={styles.container}> <Text style={styles.title}>Simple MultiSlider</Text> <MultiSlider values={[simpleValue]} onValuesChange={handleSimpleSliderChange} sliderLength={300} min={0} max={100} step={1} allowOverlap={false} snapped={true} /> <Text style={styles.title}>Two-Way MultiSlider</Text> <MultiSlider values={twoWayValue} onValuesChange={handleTwoWaySliderChange} sliderLength={300} min={0} max={100} step={1} allowOverlap={false} snapped={true} markerStyle={{ backgroundColor: 'green' }} selectedStyle={{ backgroundColor: 'green' }} unselectedStyle={{ backgroundColor: 'silver' }} /> <Text style={styles.title}>Custom MultiSlider</Text> <MultiSlider values={customValue} onValuesChange={handleCustomSliderChange} sliderLength={300} min={0} max={100} step={1} allowOverlap={false} snapped={true} customMarker={props => { return ( <View style={styles.customMarker}> <Text style={styles.customMarkerText}>{props.currentValue}</Text> </View> ); }} customMarkerLeft={props => { return ( <View style={styles.customMarker}> <Text style={styles.customMarkerText}>{props.currentValue}</Text> </View> ); }} customMarkerRight={props => { return ( <View style={styles.customMarker}> <Text style={styles.customMarkerText}>{props.currentValue}</Text> </View> ); }} selectedStyle={{ backgroundColor: 'green' }} unselectedStyle={{ backgroundColor: 'silver' }} /> </View> ); }; const styles = StyleSheet.create({ container: { flex: 1, alignItems: 'center', justifyContent: 'center', backgroundColor: '#f5fcff', }, title: { fontSize: 20, fontWeight: 'bold', marginVertical: 10, }, customMarker: { backgroundColor: 'green', borderRadius: 50, borderWidth: 1, borderColor: 'green', height: 40, width: 40, justifyContent: 'center', alignItems: 'center', }, customMarkerText: { color: 'white', fontWeight: 'bold', }, }); export default App;
Coming Soon…
Quick Links
Legal Stuff