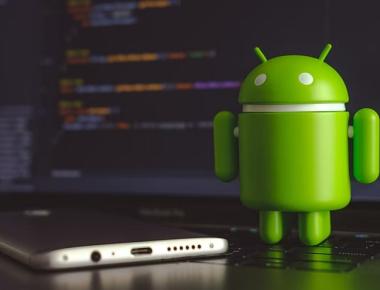
Deep Linking in React Native: What it is and How to Implement it
As mobile applications become more complex, developers are constantly seeking ways to improve the user experience. One feature that has gained popularity in recent years is deep linking. In this article, we will explore what deep linking is, why it is important, and how to implement it in a React Native application.
Deep linking is the practice of linking directly to specific pages or content within a mobile application. It enables users to access specific parts of an application without having to navigate through the app from the start. For example, a user could receive a link via email or social media that takes them directly to a specific product page within an e-commerce app, instead of having to navigate to the product manually.
Deep links can be implemented using either custom URI schemes or universal links. Custom URI schemes use a unique protocol that is specific to the app, while universal links use a website domain and can be shared across multiple apps. Both methods can be used to create deep links, but universal links are recommended as they provide a better user experience and can be easily tracked using analytics.
Deep linking has several benefits for both developers and users. From a developer’s perspective, it can increase user engagement and retention by making it easier for users to access specific content within the app. It can also improve the app’s discoverability by allowing users to find specific content through search engines or social media.
From a user’s perspective, deep linking can save time and frustration by allowing them to easily access the content they are interested in. It can also improve the user experience by allowing them to seamlessly switch between different apps or websites. For example, a user could be browsing a news article in a mobile app, click on a link to a related video, and be taken directly to the video within a video streaming app.
Implementing deep linking in a React Native application can be done using the react-native-deep-linking library. This library provides a simple API for handling incoming deep links and navigating to the correct screen within the app.
Here’s how to implement deep linking in a React Native application:
Install the react-native-deep-linking library:
npm install react-native-deep-linking --save
Define the deep link routes in your app by creating a JavaScript object that maps the deep link URL to the screen that should be displayed:
const deepLinkRoutes = {'/product/:productId': {screen: 'ProductScreen',},'/settings': {screen: 'SettingsScreen',},};
Add the DeepLinking component to the root of your app:
import { DeepLinking } from 'react-native-deep-linking';const App = () => {return (<View><DeepLinking routes={deepLinkRoutes} /><NavigationContainer>...</NavigationContainer></View>);};
Handle the incoming deep links in the componentDidMount method of the screen that should be displayed:
import { useLinking } from '@react-navigation/native';const ProductScreen = () => {const { getInitialState } = useLinking(ref, {prefixes: ['myapp://'],config: {ProductScreen: '/product/:productId',},});useEffect(() => {getInitialState().then((state) => {if (state) {navigation.reset({index: 0,routes: [{ name: state.routes[0
Add the deep link URL to your app’s share content:
Share.share({title: 'My App',message: 'Check out this product: myapp://product/123',});
With these steps, your React Native app is now capable of handling deep links. When a user clicks on a deep link, the DeepLinking component will parse the URL and navigate to the appropriate screen within the app. Conclusion
Deep linking is a powerful feature that can improve the user experience of a mobile application. By allowing users to access specific content within an app with just one click, deep linking can increase user engagement, retention, and discoverability. Implementing deep linking in a React Native app is a simple process that can be done using the react-native-deep-linking library. By following the steps outlined in this article, you can easily enable deep linking in your React Native app and improve the user experience.
Quick Links
Legal Stuff