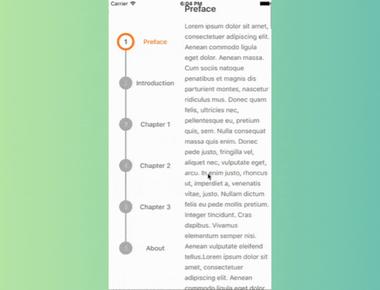
React Native Gifted Chat is a popular open-source library that provides a simple and customizable chat interface for mobile apps. It is built on top of React Native, a JavaScript framework used for building native mobile applications, and provides an easy way to implement a chat feature in your app.
In this article, we will explore the basics of using React Native Gifted Chat to create a chat interface in your app.
To get started with React Native Gifted Chat, you first need to install it as a dependency in your project. You can do this by running the following command in your terminal:
javaCopy code
npm install react-native-gifted-chat
Once you have installed the library, you can start setting up the chat component. To create a chat interface using Gifted Chat, you need to define a few basic components:
The GiftedChat
component is the main component provided by the library that brings all these components together.
import { GiftedChat } from "react-native-gifted-chat"; class ChatScreen extends React.Component { state = { messages: [] }; onSend(messages = []) { this.setState((previousState) => ({ messages: GiftedChat.append(previousState.messages, messages), })); } render() { return ( <GiftedChat messages={this.state.messages} onSend={(messages) => this.onSend(messages)} user={{ _id: 1 }} /> ); } }
In the above example, we define a ChatScreen
component that initializes the state
with an empty array of messages
. The onSend
function is used to update the state
with the new message that was sent by the user.
We then render the GiftedChat
component with the following props:
messages
: An array of messages that are displayed in the chat interface.onSend
: A callback function that is called when the user sends a message.user
: An object that represents the user that is currently using the chat.With this basic setup, you can already start testing your chat interface.
One of the benefits of using React Native Gifted Chat is that it provides a wide range of customization options. You can change the style of the chat bubbles, add extra buttons to the input bar, or even implement your own message rendering component.
Here are some examples of customization options that you can use:
<GiftedChat ... renderBubble={props => { return ( <Bubble {...props} wrapperStyle={{ right: { backgroundColor: '#2e64e5', }, }} /> ); }} />
<GiftedChat ... renderActions={props => { return ( <CustomActions {...props} /> ); }} />
<GiftedChat ... renderMessage={props => { return ( <CustomMessage {...props} /> ); }} />
By using these customization options, you can create a chat interface that fits your app’s design and functionality requirements.
React Native Gifted Chat is a powerful library that makes it easy to implement a chat interface in your app. With its simple and customizable components, you can create a chat interface that fits your app’s
Quick Links
Legal Stuff