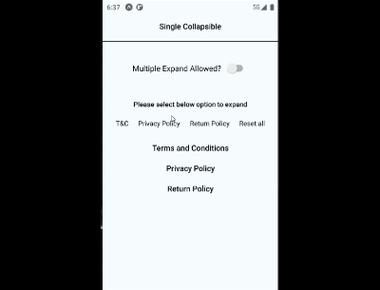
Single, Multiple Accordion With Switch
June 13, 2022
1 min
Animated collapsible component for React Native using the new Animated API with fallback. Good for accordions, toggles etc
npm install --save react-native-collapsible
and
npm install --save react-native-collapsible-accordion
and
npm install --save react-native-animatable
import React, { Component } from 'react'; import { Switch, ScrollView, StyleSheet, Text, View, TouchableOpacity, } from 'react-native'; import Constants from 'expo-constants'; import * as Animatable from 'react-native-animatable'; import Collapsible from 'react-native-collapsible'; import Accordion from 'react-native-collapsible/Accordion'; const BACON_IPSUM = 'Bacon ipsum dolor amet chuck turducken landjaeger tongue spare ribs. Picanha beef prosciutto meatball turkey shoulder shank salami cupim doner jowl pork belly cow. Chicken shankle rump swine tail frankfurter meatloaf ground round flank ham hock tongue shank andouille boudin brisket. '; const CONTENT = [ { title: 'First', content: BACON_IPSUM, }, { title: 'Second', content: BACON_IPSUM, }, { title: 'Third', content: BACON_IPSUM, }, { title: 'Fourth', content: BACON_IPSUM, }, { title: 'Fifth', content: BACON_IPSUM, }, ]; export default class App extends Component { state = { activeSections: [], collapsed: true, multipleSelect: false, }; toggleExpanded = () => { this.setState({ collapsed: !this.state.collapsed }); }; setSections = (sections) => { this.setState({ activeSections: sections.includes(undefined) ? [] : sections, }); }; renderHeader = (section, _, isActive) => { return ( <Animatable.View duration={400} style={[styles.header, isActive ? styles.active : styles.inactive]} transition="backgroundColor" > <Text style={styles.headerText}>{section.title}</Text> </Animatable.View> ); }; renderContent(section, _, isActive) { return ( <Animatable.View duration={400} style={[styles.content, isActive ? styles.active : styles.inactive]} transition="backgroundColor" > <Text>{section.content}</Text> </Animatable.View> ); } render() { const { multipleSelect, activeSections } = this.state; return ( <View style={styles.container}> <ScrollView contentContainerStyle={{ paddingTop: 30 }}> <View style={styles.multipleToggle}> <Text style={styles.multipleToggle__title}>Accordion</Text> </View> <Collapsible collapsed={this.state.collapsed}> <View style={styles.content}> <Animatable.Text animation={this.state.collapsed ? undefined : 'zoomIn'} duration={300} useNativeDriver > Bacon ipsum dolor amet chuck turducken landjaeger tongue spare ribs </Animatable.Text> </View> </Collapsible> <Accordion align="bottom" activeSections={activeSections} sections={CONTENT} touchableComponent={TouchableOpacity} expandMultiple={multipleSelect} renderHeader={this.renderHeader} renderContent={this.renderContent} duration={400} onChange={this.setSections} renderAsFlatList={false} /> </ScrollView> </View> ); } } const styles = StyleSheet.create({ container: { flex: 1, backgroundColor: '#F5FCFF', paddingTop: Constants.statusBarHeight, }, title: { textAlign: 'center', fontSize: 22, fontWeight: '300', marginBottom: 20, }, header: { backgroundColor: '#F5FCFF', padding: 10, }, headerText: { textAlign: 'center', fontSize: 16, fontWeight: '500', }, content: { padding: 20, backgroundColor: '#fff', }, active: { backgroundColor: 'rgba(255,255,255,1)', }, inactive: { backgroundColor: 'rgba(245,252,255,1)', }, selectors: { marginBottom: 10, flexDirection: 'row', justifyContent: 'center', }, selector: { backgroundColor: '#F5FCFF', padding: 10, }, activeSelector: { fontWeight: 'bold', }, selectTitle: { fontSize: 14, fontWeight: '500', padding: 10, }, multipleToggle: { flexDirection: 'row', justifyContent: 'center', marginVertical: 30, alignItems: 'center', }, multipleToggle__title: { fontSize: 16, marginRight: 8, }, });
Quick Links
Legal Stuff