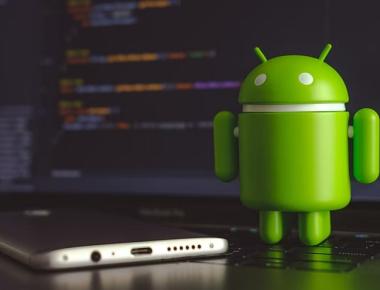
React Native Color Picker is a third-party library that allows you to add a color picker to your React Native app. It offers both uncontrolled and controlled components that you can use to let the user select a color from a color wheel. The ColorPicker component is the uncontrolled version of the color picker, and it has the following props:
• defaultColor: The default color of the color picker. If this prop is not set, the color picker will use black as the default color. • oldColor: The initial color of the color picker. If this prop is set, the color picker will show a preview of the old color, and the user will be able to revert to it by clicking on the “Old” button. • onColorChange: A function that will be called every time the user changes the color. The function will receive the new color as a string in the format “#RRGGBB”. • onColorSelected: A function that will be called when the user selects a color. The function will receive the selected color as a string in the format “#RRGGBB”.
Color pickers accepts properties below. Each property which define color is represented as a color string.
Both color pickers are PureComponents thus if you want to update it you should not mutate its properties deeply.
Property | Type | Note |
---|---|---|
color | String\|HSV | Color string or HSV object (see below). Defines selected color in controlled component. |
defaultColor | String | Defines initial selected color in uncontrolled component. |
oldColor | String | Old color to be used for visual comparision. If it is not defined, whole circle is representing selected color. |
style | Style | Styles passed to color picker container |
onColorSelected | Function | Callback with color (HEX string) as argument called when user confirms color selection. |
onColorChange | Function | Callback called each time when color is changed. Used in controlled component. Argument is color in HSV representation (see below) |
onOldColorSelected | Function | Callback with color (HEX string) as argument called when user selects old color. |
hideSliders | Boolean | Option to hide bottom sliders (holo picker only) |
hideControls | Boolean | Option to hide bottom buttons (triangle picker only) |
npm install react-native-color-picker --save
And other required dependencies
npm install react-native-paper
npm install @expo/vector-icons
import React from 'react' import { StyleSheet, View, Text} from 'react-native' import { TriangleColorPicker, toHsv } from 'react-native-color-picker' class App extends React.Component { constructor(...args) { super(...args) this.state = { example: null } } onColorChange(color) { this.setState({ color }) } render() { return ( <View style={styles.container}> <Text style={{color: 'white'}}>React Native Color Picker - Controlled</Text> <TriangleColorPicker oldColor='purple' color={this.state.color} onColorChange={this.onColorChange} onColorSelected={color => alert(`Color selected: ${color}`)} onOldColorSelected={color => alert(`Old color selected: ${color}`)} style={{flex: 1}} /> </View> ) } } const styles = StyleSheet.create({ container: { flex: 1, padding: 45, backgroundColor: '#212021', }, }) export default App
Coming Soon…
Quick Links
Legal Stuff