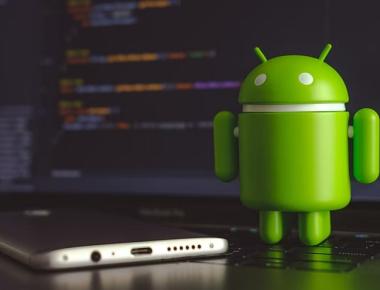
AdMob is a popular mobile advertising platform that enables developers to monetize their mobile applications by displaying ads. React Native is a widely-used framework for building mobile apps using JavaScript and is compatible with AdMob. In this article, we will explore the steps required to integrate AdMob into a React Native application.
Step 1: Create a new React Native application
The first step is to create a new React Native application using the following command:
npx react-native init AdMobExample
This command will create a new React Native project named “AdMobExample”.
Step 2: Install the required dependencies
Next, you need to install the required dependencies for integrating AdMob into your React Native application. You can do this using the following command:
npm install react-native-google-mobile-ads --save
This command will install the “react-native-google-mobile-ads” package, which is a third-party library that enables you to integrate AdMob into your React Native application.
Step 3: Create an AdMob account
To use AdMob, you need to create an AdMob account. You can do this by visiting the AdMob website and following the steps to sign up. Once you have created an AdMob account, you can create an ad unit, which will provide you with an ad unit ID that you will use to display ads in your application.
Step 4: Configure AdMob in your React Native application
To configure AdMob in your React Native application, you need to add the following code to your “index.js” file:
import { AppRegistry } from 'react-native';import { AdMobInterstitial } from 'react-native-google-mobile-ads';AdMobInterstitial.setAdUnitID('your-ad-unit-id');AdMobInterstitial.setTestDevices([AdMobInterstitial.simulatorId]);AdMobInterstitial.requestAd().then(() => AdMobInterstitial.showAd());
This code imports the “AdMobInterstitial” component from the “react-native-admob” package, sets the ad unit ID to your ad unit ID, sets the test device to the simulator, requests an ad, and then shows the ad.
Step 5: Display an AdMob ad in your React Native application
To display an AdMob ad in your React Native application, you need to add the following code to your application:
import React, { Component } from 'react';import { View, Text } from 'react-native';import { AdMobBanner } from 'react-native-admob';export default class App extends Component {render() {return (<View><Text>Welcome to AdMobExample</Text><AdMobBanneradSize="fullBanner"adUnitID="your-ad-unit-id"testDevices={[AdMobBanner.simulatorId]}onAdFailedToLoad={error => console.error(error)}/></View>);}}AppRegistry.registerComponent('AdMobExample', () => App);
This code imports the “AdMobBanner” component from the “react-native-google-mobile-ads” package and displays an ad using the ad unit ID and test device set in the previous step.
Step 6: Test your AdMob integration
To test your AdMob integration, you can run your React Native application in the simulator or on a device. If everything is set up correctly, you should see an AdMob ad displayed in your application.
Quick Links
Legal Stuff