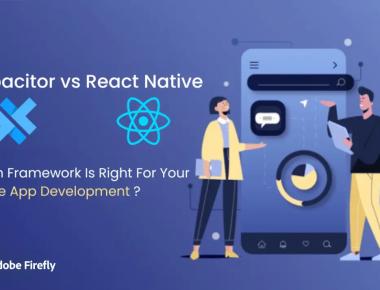
React Native is a popular framework for building native mobile applications using JavaScript and React. One of the key benefits of React Native is that it allows developers to use platform-specific components and APIs, which can improve the performance and user experience of the application. One such component is the WebView, which allows developers to embed web content into their application. In this article, we’ll explore how to use the WebView component in React Native.
Before we get started, make sure that you have the following tools installed on your machine:
To get started, create a new React Native project using the following command:
javaCopy code
npx react-native init WebViewExample
This will create a new React Native project called WebViewExample
.
In the App.js
file, import the WebView
component from react-native
and add it to the render method as follows:
import React, { Component } from 'react'; import { View, WebView } from 'react-native'; class App extends Component { render() { return ( <View style={{ flex: 1 }}> <WebView source={{ uri: 'https://google.com' }} /> </View> ); } } export default App;
This will add a WebView component to the app and load the Google website in it.
The WebView component provides several props that allow you to control the behavior of the WebView. For example, you can use the onLoad
prop to be notified when the WebView has finished loading, and the onError
prop to handle any errors that occur while loading the content.
import React, { Component } from 'react'; import { View, WebView } from 'react-native'; class App extends Component { handleLoad = () => { console.log('WebView loaded successfully'); }; handleError = (error) => { console.log('WebView error:', error); }; render() { return ( <View style={{ flex: 1 }}> <WebView source={{ uri: 'https://google.com' }} onLoad={this.handleLoad} onError={this.handleError} /> </View> ); } } export default App;
In the example above, we’re logging a message to the console when the WebView has finished loading or encounters an error.
The WebView component also provides a way to communicate between the web content and the React Native app using the onMessage
prop. This allows you to send messages from the web content to the React Native app and vice versa.
import React, { Component } from 'react'; import { View, WebView } from 'react-native'; class App extends Component { handleLoad = () => { console.log('WebView loaded successfully'); }; handleMessage = (event) => { const { data } = event.nativeEvent; console.log('Received message from WebView:', data); }; sendMessage = () => { this.webView.postMessage('Hello from React Native!'); }; render() { return ( <View style={{ flex: 1 }}> <WebView ref={(webView) => (this.webView = webView)} source={{ uri: 'https://google.com' }} onLoad={this.handleLoad} onMessage={this.handleMessage} /> <Button title="Send Message" onPress={this.sendMessage} /> </View>
Quick Links
Legal Stuff