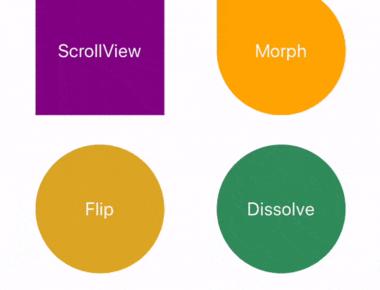
Reanimated is a React Native library that allows for creating smooth animations and interactions that runs on the UI thread.
In React Native apps, the application code is executed outside of the application main thread. This is one of the key elements of React Native architecture and helps with preventing frame drops in cases where JavaScript has some heavy work to do. Unfortunately this design does not play well when it comes to event driven interactions. When interacting with a touch screen, the user expects effects on screen to be immediate. If updates are happening in a separate thread it is often a case that changes done in the JavaScript thread cannot be reflected in the same frame. In React Native by default all updates are delayed by at least one frame as the communication between UI and JavaScript thread is asynchronous and UI thread never waits for the JavaScript thread to finish processing events. On top of the lag with JavaScript playing many roles like running react diffing and updates, executing app’s business logic, processing network requests, etc., it is often the case that events can’t be immediately processed thus causing even more significant delays. Reanimated aims to provide ways of offloading animation and event handling logic off of the JavaScript thread and onto the UI thread. This is achieved by defining Reanimated worklets – a tiny chunks of JavaScript code that can be moved to a separate JavaScript VM and executed synchronously on the UI thread. This makes it possible to respond to touch events immediately and update the UI within the same frame when the event happens without worrying about the load that is put on the main JavaScript thread.
Installing Reanimated requires a couple of additional steps compared to installing most of the popular react-native packages. Specifically on Android the setup consist of adding additional code to the main application class. The steps needed to get reanimated properly configured are listed in the below paragraphs. Installing the package
First step is to install react-native-reanimated as a dependency in your project:
yarn add react-native-reanimated
Add Reanimated’s babel plugin to your babel.config.js:
module.exports = { ... plugins: [ ... 'react-native-reanimated/plugin', ], };
<Button onPress={() => { offset.value = withSpring(Math.random()); }} title="Move" />
import Animated, { withSpring, useAnimatedStyle, useSharedValue, } from 'react-native-reanimated'; function Box() { const offset = useSharedValue(0); const defaultSpringStyles = useAnimatedStyle(() => { return { transform: [{ translateX: withSpring(offset.value * 255) }], }; }); const customSpringStyles = useAnimatedStyle(() => { return { transform: [ { translateX: withSpring(offset.value * 255, { damping: 20, stiffness: 90, }), }, ], }; }); return ( <> <Animated.View style={[styles.box, defaultSpringStyles]} /> <Animated.View style={[styles.box, customSpringStyles]} /> <Button onPress={() => (offset.value = Math.random())} title="Move" /> </> ); }
const EventsExample = () => { const pressed = useSharedValue(false); return ( <TapGestureHandler onGestureEvent={eventHandler}> <Animated.View style={[styles.ball]} /> </TapGestureHandler> ); };
Make sure to install the react-native-gesture-handler
for usage.
Quick Links
Legal Stuff