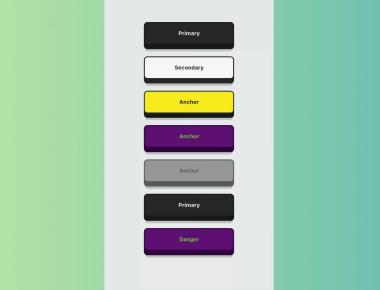
Custom Button 2
May 06, 2023
1 min
Props | Description | Default |
---|---|---|
style | button style -width : width of button -height :height of button. -borderWidth :width of outer border. -borderRadius : radius of outer border, this will influence inner progress view in android. -backgroundColor : background color of button, not visiable when buttonState is ‘static’. -padding : padding area betweenn outer border and inner progresss view | -width :400 -height :40 -borderWidth :0 -borderRadius :5-backgroundColor :‘limegreen’-padding :0 |
buttonState | the state which control button whether in progress, one of three follow value:-'staic' : static button, button not in progress.-'indeterminate' : indeterminate progress button, activityIndicator is shown.-'progress' : like progress bar. | 'static' |
smoothly | whether the progress is smooth,only used when buttonState is 'progress' | true |
paused | Whether to pause the animation of progress,-false : pause a progress animation or deley new progress animation start.-true : restart a progress animation, The progress speed is the same as before the pause, so try not set timingConfig before restart the progress | false |
timingConfig | config of the Animated.timing() in which smooth progress animation used. duration , delay , easing | {duration: 100} |
progressColor | background color of inner progress bar. | 'limegreen' |
unfilledColor | Color of the remaining progress. | 'lightgrey' |
progress | Progress value when the button in ‘progress’ state. A number between 0 and maxProgress | 0 |
activityIndicator | a indetermimate indicator when buttonState is 'indetermimate' ,shown left of text | ActitvityIndicator |
activityIndicatorPadding | padding area between indetermimate indicator and text | 5 |
text | Text shown in the center of the button | ‘OK’ |
textStyle | text style of the text. they will be included in style attr of text | textStyle:{color: 'white'} |
onPress | A function to be called as soon as the user press the button.(event, buttonState, progress) => {} | |
onProgressAnimatedFinished | A function to be called as soon as the progress animation finished, (progress) => {} |
npm install react-native-progress-button --save
import React from 'react'; import {View, StyleSheet, Text, processColor, Image} from 'react-native'; // import ProgressButton from './src/ProgressButton'; import {ProgressButton} from 'react-native-progress-button'; const styles = StyleSheet.create({ container: { flex:1, alignItems: 'center', paddingTop: 40 }, progressContainer: { flexDirection:'column', alignItems:'center', marginTop:20 }, spinProgressButton: { width: 100, height: 20, }, }); class App extends React.Component{ constructor(props) { super(props); this.state = { continueProgressButton:{ text:'Tap Me', }, timerButton:{ text:'Tap Me', }, customStyleButton:{ style: { width:240, height: 50, borderRadius: 25, borderColor: 'blue', borderWidth:2, backgroundColor: 'red', padding:2, }, progressColor:'white', unfilledColor:'grey', text:'Tab Me', textStyle: { color:'green', fontSize: 12, fontStyle:'italic' } } } } onContinuePress = (event, buttonState, progress) => { console.log('onContinuePress', buttonState, progress); if (buttonState === 'static') { this.setState((prevState) => { return Object.assign({}, prevState, { continueProgressButton:{ ...prevState.continueProgressButton, buttonState: 'indeterminate' } }) }) } if (buttonState === 'indeterminate') { this.setState((prevState) => { return Object.assign({}, prevState, { continueProgressButton: { ...prevState.continueProgressButton, progress: 10, buttonState:'progress', text: 'Press to add progress:' + 10 + '%' } }) }); } if (buttonState === 'progress' && progress > 0 && progress < 100) { this.setState((prevState) => { return Object.assign({}, prevState, { continueProgressButton: { ...prevState.continueProgressButton, progress: Math.min(progress + 20, 100), text: 'Press to add progress:' + Math.min(progress + 20, 100) + '%' } }) }) } if (buttonState === 'progress' && progress >= 100) { this.setState((prevState) => { return Object.assign({}, prevState, { continueProgressButton: { ...prevState.continueProgressButton, text: 'Completed' } }) }) } }; onTimerLoadingPress = (event, buttonState, progress) => { if (buttonState === 'static') { console.log('onTimerLoadingPress-restart'); this.setState({ timerButton: { timingConfig:{ duration:5000, }, buttonState:'progress', progress:100, text:'Timing', paused: false } }) } if (buttonState === 'progress') { this.setState((prevState) => { return { timerButton: { ...this.state.timerButton, paused: !prevState.timerButton.paused, text: prevState.timerButton.paused? 'Timing' : 'Restart' } }; }); } }; onTimerFinished = (progress)=> { if (progress === 100) { this.setState({ timerButton: { ...this.timerButton, text:'Completed' } }); } }; onCustomButtonPress = (event, buttonState) => { if (buttonState === 'static') { this.setState((prevState) => { return Object.assign({}, prevState, { customStyleButton:{ ...prevState.customStyleButton, buttonState:'indeterminate', progress:0 } }) }) } if (buttonState === 'indeterminate') { this.setState((prevState) => { return Object.assign({}, prevState, { customStyleButton:{ ...prevState.customStyleButton, timingConfig:{ duration:5000, }, buttonState:'progress', progress:100, text:'Timing', paused: false } }) }) } if (buttonState === 'progress') { this.setState((prevState) => { return Object.assign({}, prevState, { customStyleButton:{ ...prevState.customStyleButton, buttonState:'static', } }) }) } }; customIndicator = <Image style={{width: 20, height: 20}} source={require('./src/resource/loading.gif')}></Image>; render() { return ( <View style={styles.container}> <View style={styles.progressContainer}> <Text>Continue Progress Button</Text> <ProgressButton {...this.state.continueProgressButton} onPress={this.onContinuePress.bind(this)}/> </View> <View style={styles.progressContainer}> <Text>Timer Button</Text> <ProgressButton {...this.state.timerButton} onPress={this.onTimerLoadingPress.bind(this)} onProgressAnimatedFinished={this.onTimerFinished.bind(this)} paused={this.state.timerButton.paused}/> </View> <View style={styles.progressContainer}> <Text>Custom Style Button</Text> <ProgressButton {...this.state.customStyleButton} activityIndicator={this.customIndicator} onPress={this.onCustomButtonPress.bind(this)} /> </View> </View> ); } } export default App;
Coming Soon…
Quick Links
Legal Stuff