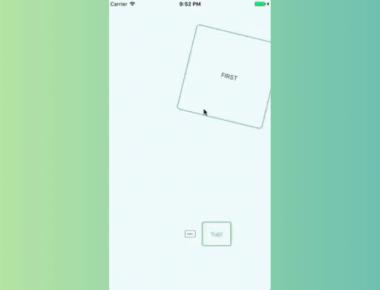
React Native Swiper Animated is a lightweight, customizable, and easy-to-use component that provides smooth and beautiful animations for image and content swiping in React Native. Here’s a brief guide on how to use it.
react-native-swiper-animated provides a wide range of customizable props to control the behavior and appearance of the component: • style: Style object for the wrapper view of the swiper. • containerStyle: Style object for the container view of each slide. • cardWidth: Width of each slide. Defaults to 250. • cardHeight: Height of each slide. Defaults to 250. • cardBorderRadius: Border radius of each slide. Defaults to 8. • cardPadding: Padding of each slide. Defaults to 16. • cardMargin: Margin of each slide. Defaults to 16. • cardHorizontalMargin: Horizontal margin of each slide. Defaults to 16. • cardVerticalMargin: Vertical margin of each slide. Defaults to 16. • cardSpacing: Spacing between each slide. Defaults to 16. • cardElevation: Elevation of each slide. Defaults to 2. • cardOpacity: Opacity of each slide. Defaults to 1. • cardAnimationDuration: Duration of the slide animation. Defaults to 500. • cardAnimationEasing: Easing function of the slide animation. Defaults to Easing.quad. • cardAnimationDelay: Delay of the slide animation. Defaults to 0. • index: Index of the current slide. • animatedValue: Animated value used for the slide animation. • onChangeIndex: Function called when the current slide changes. • showPagination: Whether to show the pagination indicators. Defaults to true. • paginationActiveColor: Color of the active pagination indicator. Defaults to #007aff. • paginationInactiveColor: Color of the inactive pagination indicators. Defaults to #dcdcdc. • paginationContainerStyle: Style object for the pagination container view. • paginationStyle: Style object for the pagination indicator view. • paginationItemStyle: Style object for the pagination item view. • paginationItemOpacity: Opacity of the pagination item. Defaults to 0.6.
To use react-native-swiper-animated, you first need to install it as a dependency in your React Native project. You can install it using npm or yarn:
npm install react-native-swiper-animated
or
yarn add react-native-swiper-animated
import React, { Component } from 'react'; import { View, StyleSheet, Image, Text } from 'react-native'; import Swiper from 'react-native-swiper-animated'; class App extends Component { render() { return ( <View style={styles.container}> <Swiper style={styles.wrapper} showsButtons={false} loop={false} paginationStyle={styles.pagination} dot={<View style={styles.dot} />} activeDot={<View style={styles.activeDot} />} > <View style={styles.slide}> <Image source={{ uri: 'https://picsum.photos/id/10/400/600' }} style={styles.image} /> <Text style={styles.text}>Slide 1</Text> </View> <View style={styles.slide}> <Image source={{ uri: 'https://picsum.photos/id/20/400/600' }} style={styles.image} /> <Text style={styles.text}>Slide 2</Text> </View> <View style={styles.slide}> <Image source={{ uri: 'https://picsum.photos/id/30/400/600' }} style={styles.image} /> <Text style={styles.text}>Slide 3</Text> </View> </Swiper> </View> ); } } const styles = StyleSheet.create({ container: { flex: 1, backgroundColor: '#fff', }, wrapper: {}, slide: { flex: 1, justifyContent: 'center', alignItems: 'center', backgroundColor: '#fff', }, image: { width: '100%', height: '80%', }, text: { color: '#000', fontSize: 24, fontWeight: 'bold', marginTop: 20, }, pagination: { bottom: 10, }, dot: { backgroundColor: '#ccc', width: 10, height: 10, borderRadius: 5, marginLeft: 3, marginRight: 3, marginTop: 3, marginBottom: 3, }, activeDot: { backgroundColor: '#000', width: 10, height: 10, borderRadius: 5, marginLeft: 3, marginRight: 3, marginTop: 3, marginBottom: 3, }, }); export default App;
Coming Soon…
Quick Links
Legal Stuff