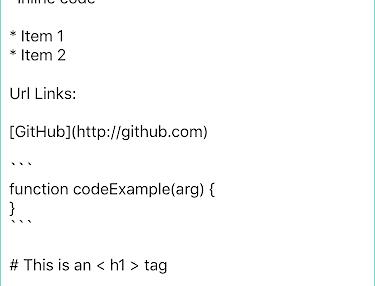
Measure text height and/or width without laying it out.
Run
$ yarn add react-native-measure-text
or, if you want the latest features, then run:
$ yarn add react-native-measure-text@next
And then:
$ react-native link react-native-measure-text
Libraries
➜ Add Files to [your project's name]
node_modules
➜ react-native-measure-text
and add RNMeasureText.xcodeproj
libRNMeasureText.a
to your project’s Build Phases
➜ Link Binary With Libraries
Cmd+R
)<android/app/src/main/java/[...]/MainActivity.java
import io.github.airamrguez.RNMeasureTextPackage;
to the imports at the top of the filenew RNMeasureTextPackage()
to the list returned by the getPackages()
methodandroid/settings.gradle
:include ':react-native-measure-text' project(':react-native-measure-text').projectDir = new File(rootProject.projectDir, '../node_modules/react-native-measure-text/android')
android/app/build.gradle
:compile project(':react-native-measure-text')
import MeasureText from 'react-native-measure-text'; const texts = [ 'This is an example', 'This is the second line' ]; const width = 100; const fontSize = 15; const fontFamily = 'Arvo'; class Test extends Component { state = { heights: [], } async componentDidMount() { const heights = await MeasureText.heights({( texts, /* texts to measure */ width, /* container width */ fontSize, fontFamily /* fontFamily is optional! */ ); this.setState({ heights }); } render() { const { heights } = this.state; return ( <View> {texts.map((text, i) => ( <Text key={`text-${i}`} style={{ width, fontSize, fontFamily, height: heights[i], }} > {text} </Text> ))} </View> } }
MeasureText.heights(options)
Returns a promise that resolves to all the heights of the texts passed in options.
MeasureText.widths(options)
Returns a promise that resolves to all the widths of the texts passed in options.
Measure options:
texts
: An array of texts to measure.width
: Container width when you want to measure the height.height
: Container height when you want to measure the width.fontSize
: The size of the font.fontFamily
: The name of a custom font or a preinstalled font. This is optional.fontWeight
: Specifies font weight. The values are the same that React Native allows: enum('normal', 'bold', '100', '200', '300', '400', '500', '600', '700', '800', '900')
Follow these steps:
assets/fonts
at the root of your React Native project.package.json
file:"rnpm": { "assets": [ "./assets/fonts/" ] }
react-native link
on the root of your project to link the added fonts.fontWeight
If you are using custom fonts then you have to add the bold version into the assets/fonts
directory. Follow the convention that React Native applies. Extracted from the docs:
Given a “family” font family the files in the assets/fonts folder need to be family.ttf (.otf) family_bold.ttf(.otf) family_italic.ttf(.otf) and family_bold_italic.ttf(.otf)
You’re ready to go!
Quick Links
Legal Stuff